Python Pandas is an essential tool for data manipulation and analysis in Data Science. It simplifies data handling and offers powerful data structures.
Pandas is a versatile and widely-used Python library that aids in data analysis and manipulation. It provides DataFrame and Series, which are robust data structures for handling data efficiently. With Pandas, you can perform data cleaning, filtering, and transformation tasks seamlessly.
Its integration with other libraries like NumPy and Matplotlib enhances its functionality. Data scientists rely on Pandas for preprocessing and analyzing large datasets. The library’s ease of use and extensive documentation make it accessible even for beginners. Whether you’re conducting exploratory data analysis or preparing data for machine learning, Pandas proves to be an invaluable asset in your data science toolkit.
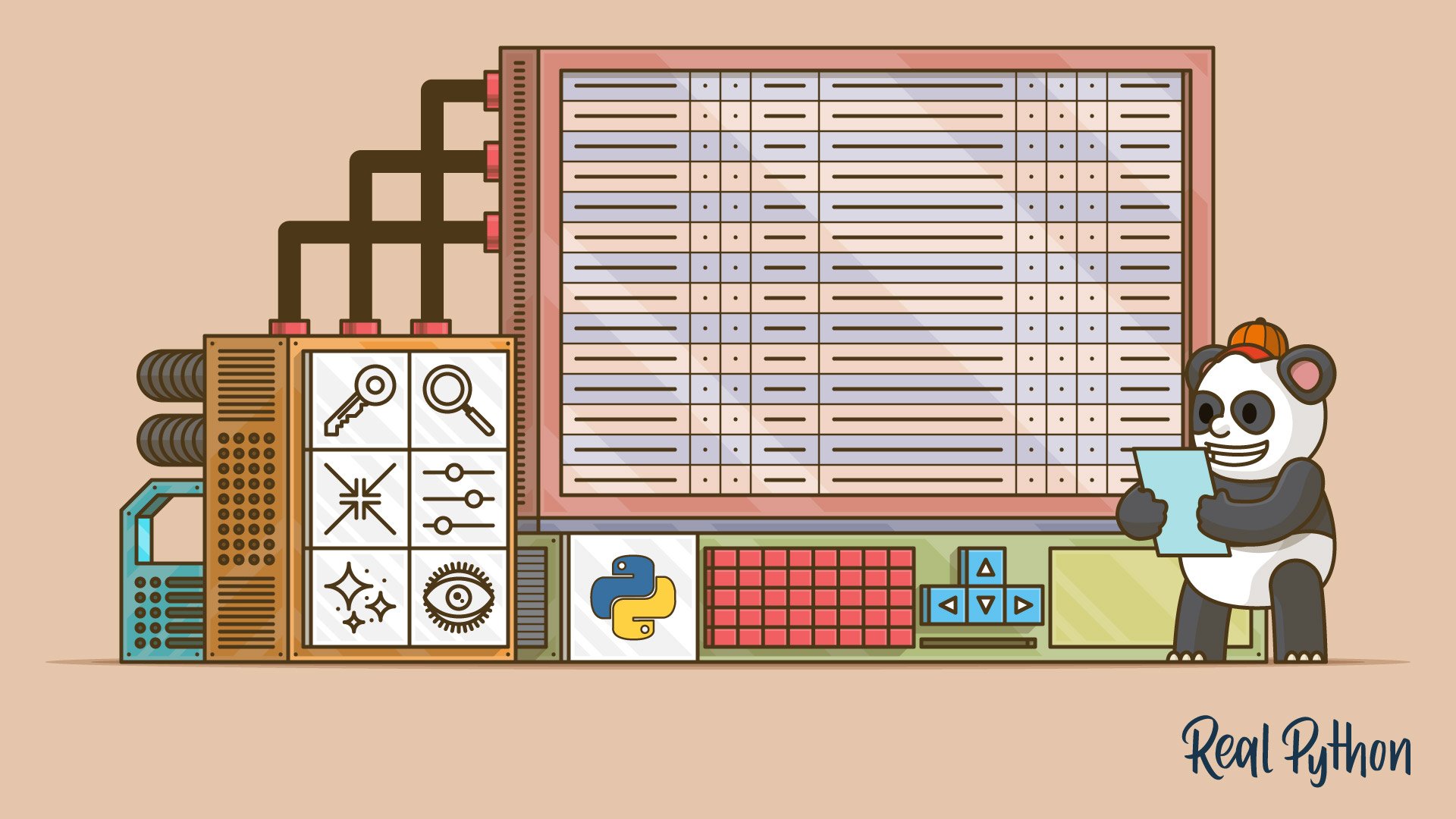
Credit: realpython.com
Introduction To Python Pandas
Python Pandas helps in handling large datasets easily. It provides powerful data structures. These structures make data analysis fast and simple. Pandas can read and write data from many sources like CSV, Excel, and SQL databases. Data cleaning becomes easy with Pandas. It can handle missing values and duplicate data. Pandas also supports data visualization with built-in functions. This makes it easier to understand data patterns.
Feature | Description |
---|---|
DataFrame | A 2-dimensional labeled data structure |
Series | A 1-dimensional labeled array |
Data Alignment | Automatically aligns data based on labels |
Reshaping | Easy reshaping and pivoting of datasets |
Data Cleaning | Handles missing and duplicate data |
Data Aggregation | Provides tools for data aggregation and grouping |
Getting Started With Pandas
Pandas is a powerful tool for data science. First, install it using pip. Type `pip install pandas` in your command line. This will download and install Pandas on your computer. Ensure you have Python installed before you start. Pandas depends on NumPy, so it will be installed too. Verify the installation by typing `import pandas as pd` in a Python script. If no error shows, the installation is successful.
Start by importing Pandas. Use `import pandas as pd`. Then, create a DataFrame to hold your data. A DataFrame is like a table. You can create it from a dictionary. For example, `data = {‘name’: [‘Alice’, ‘Bob’], ‘age’: [25, 30]}`. Convert it to a DataFrame using `df = pd.DataFrame(data)`. Now, you can analyze the data easily. Display the first few rows using `df.head()`. This will show the top records in your data.
Data Structures In Pandas
Python Pandas for Data Science offers versatile data structures like Series and DataFrame, streamlining data manipulation and analysis. These structures enhance efficiency and accuracy in managing large datasets.
Understanding Series
A Series is a one-dimensional data structure in Pandas. It can hold any data type such as integers, floats, and strings. Each element in a Series has a unique index. This index starts from zero. Series is like a column in a table. It is very useful for handling data. You can create a Series using the `pd.Series()` function. The Series data structure allows quick and easy data manipulation.
Exploring Dataframes
A DataFrame is a two-dimensional data structure. It is like a table with rows and columns. Each column in a DataFrame is a Series. DataFrames are excellent for data analysis tasks. You can create DataFrames using the `pd.DataFrame()` function. This structure allows for complex operations on data. DataFrames make it easy to filter, sort, and group data.
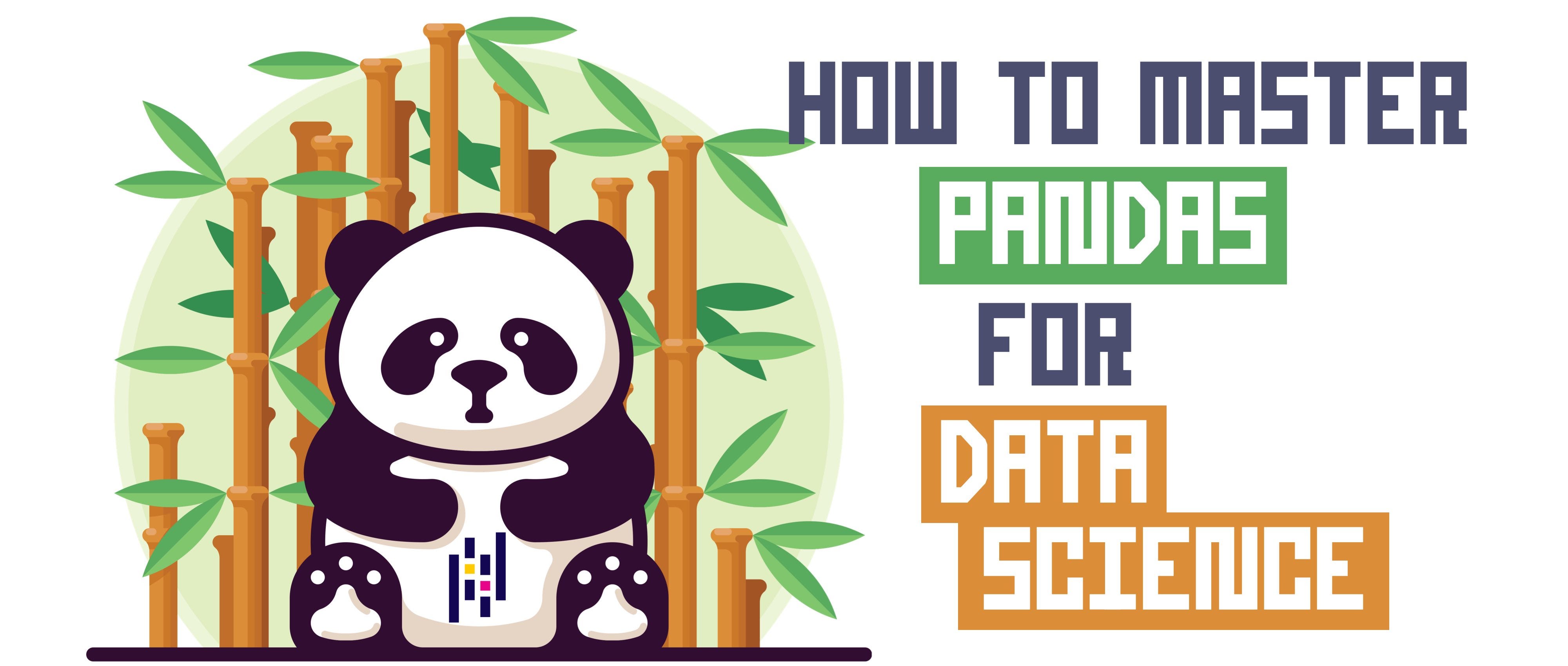
Credit: towardsdatascience.com
Data Importing Techniques
Python Pandas offers efficient data importing techniques essential for data science. Utilize functions like read_csv and read_excel to streamline data analysis tasks.
Reading Csv And Excel Files
Python Pandas can read both CSV and Excel files. Use the read_csv()
function to read CSV files. Use the read_excel()
function for Excel files. Both functions are very simple to use. Just pass the file path to them. Pandas will do the rest. This makes it easy to work with data files. CSV files are plain text files. Excel files are more complex. Pandas handles both with ease.
Importing Data From Databases
Pandas can also import data from databases. Use the read_sql()
function to do this. Connect to the database first. Then, pass the SQL query and connection to the function. Pandas will return the data in a DataFrame. This is very useful for large datasets. Databases store a lot of data. Pandas makes it easy to access this data. It simplifies the data analysis process.
Data Cleaning With Pandas
Missing values can cause problems. Use Pandas to deal with them. You can drop rows with missing values. You can also fill missing values with a default number. Another way is to use the mean or median to fill gaps. Pandas make these tasks easy and quick.
Data types must be correct. Use Pandas to convert data types. The astype() function helps to change types. You can convert strings to numbers or dates to datetime objects. Correct data types help in analysis. Pandas ensure data is in the right format.
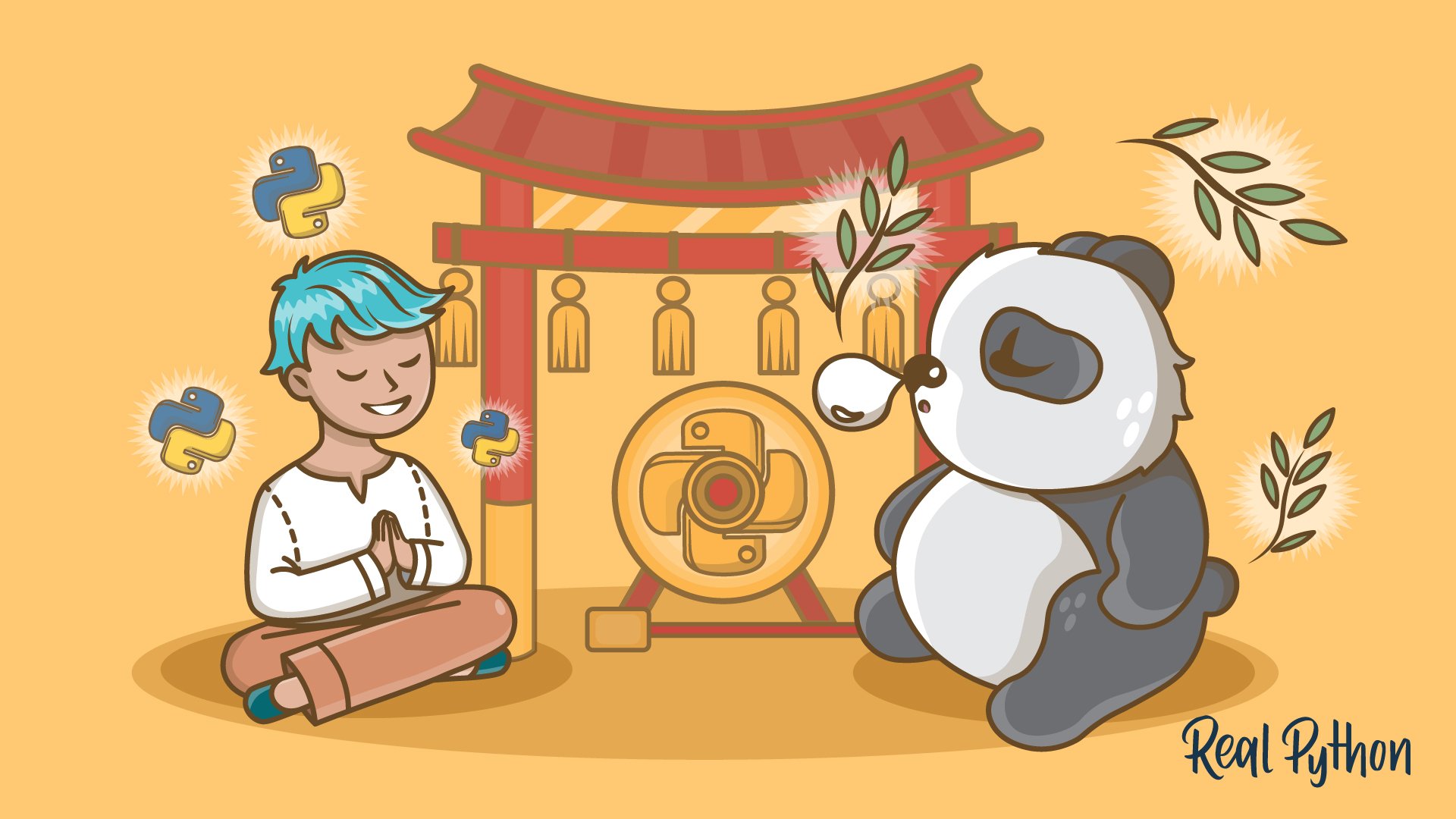
Credit: realpython.com
Data Manipulation And Transformation
Python Pandas makes data filtering easy. Use the loc and iloc functions to select rows and columns. The loc function selects data by labels. The iloc function selects data by position. You can also use boolean indexing. This helps filter data based on conditions. For example, filter rows where the value in column A is greater than 10.
Pandas makes it simple to apply functions to data. The apply function lets you apply a custom function to rows or columns. Use the agg function to perform aggregations. Aggregations include sum, mean, and count. You can also use the groupby function. This allows you to group data and then apply functions to each group.
Advanced Data Analysis
The GroupBy function in Pandas is very powerful. It helps to group data by one or more columns. You can then perform operations like sum, mean, or count on these groups. This makes it easy to understand large datasets quickly. For example, you can group sales data by region and get the total sales for each region. This provides valuable insights without much effort.
Pivot Tables are great for summarizing data. They help to create a new table that shows the data in a different way. You can use them to show the sum, average, or other metrics of your data. Cross-Tabulation is another useful feature in Pandas. It allows you to show the relationship between two different columns. For example, you can see how many products were sold in each region by month.
Data Visualization With Pandas
Python Pandas for Data Science simplifies data visualization with its powerful tools. Create insightful charts and graphs effortlessly using Pandas. Enhance data analysis by transforming complex datasets into clear visual formats.
Integrated Plotting With Matplotlib
Pandas work well with Matplotlib. It helps create beautiful charts. You can make line plots, bar charts, and more. Use the `plot()` function in Pandas. This function provides many options. Choose the type of plot you want. Pandas make data visualization easy and fun.
Creating Interactive Visuals
Interactive visuals are very engaging. Use Pandas with Plotly for this. Plotly helps create interactive plots. These plots can be zoomed and hovered over. Interactive visuals make data exploration easier. Kids can also understand better with interactive visuals.
Performance Tuning In Pandas
Use vectorized operations instead of loops. They are faster and use less memory. Avoid using apply() as much as possible. Use built-in functions like `sum()`, `mean()`, and `count()`. Breaking data into smaller chunks can also improve speed. Use the `chunksize` parameter when reading large files. This helps in processing data in manageable pieces. Remember to drop unused columns to save memory. Filtering data early helps in reducing the size of data handled. Always remember to use efficient indexing for quick data access.
Convert text data to categorical data types to save memory. This is useful for columns with repeating values. For example, gender or country columns. Use `pd.Categorical()` to convert these columns. Categorical data also speeds up operations like sorting and grouping. Categorical data types use less memory compared to object types. This can significantly boost performance. Always use categorical types for columns with limited unique values. This simple change can make a big difference in performance.
Real-world Applications Of Pandas
Pandas is used in many industries. It helps in finance to analyze stock data. In healthcare, it processes patient records. Retailers use Pandas for sales data analysis. Scientists use it to study climate data. Marketing teams analyze customer data with Pandas. It makes complex data simple to understand.
Pandas works well with machine learning. Data preprocessing is easier with Pandas. It cleans and prepares data for models. Feature engineering is faster using Pandas. Machine learning libraries like Scikit-learn use Pandas data. It helps in splitting data into training and testing sets. Data visualization is easy with Pandas and Matplotlib. It makes the machine learning process smooth.
Best Practices For Using Pandas
Use vectorized operations instead of loops. This makes your code run faster. Use the apply() function wisely. It can slow down your code. Prefer built-in functions like sum() or mean(). They are optimized for performance. Avoid chained indexing. It can lead to unexpected results.
Keep your code clean and readable. Use meaningful variable names. It helps others understand your code. Break down complex operations into smaller functions. This makes your code easier to manage. Document your code well. Use comments to explain tricky parts. This helps future developers.
Resources And Community
Discover a wealth of resources and join a vibrant community to master Python Pandas for Data Science. Enhance your skills with tutorials, forums, and expert advice.
Learning Resources
Python Pandas is a powerful tool for data science. Many online tutorials and courses teach Pandas. Websites like Coursera and Udemy offer comprehensive courses. YouTube also has many free tutorials. Books like “Python for Data Analysis” are excellent. These resources help you learn Pandas step by step. Practice is key. Try to work on small projects. This will help you understand better.
Engaging With The Pandas Community
The Pandas community is very active. Joining forums and discussion groups helps a lot. Websites like Stack Overflow and Reddit have many Pandas users. You can ask questions and share your knowledge. Follow Pandas experts on social media. They often share useful tips and updates. Attend webinars and workshops. These events are great for learning and networking.
Frequently Asked Questions
Is Pandas Useful For Data Science?
Yes, pandas is highly useful for data science. It simplifies data manipulation, analysis, and visualization tasks. This powerful library handles large datasets efficiently.
Is Numpy And Pandas Used For Data Science?
Yes, NumPy and pandas are essential tools for data science. They offer powerful data manipulation and analysis capabilities.
Is Pandas A Data Science Library?
Yes, pandas is a popular data science library. It helps in data manipulation and analysis, offering data structures and operations.
Which Python Is Best For Data Science?
Python 3. 8 or higher is best for data science. It supports popular libraries like Pandas, NumPy, and Scikit-learn.
Conclusion
Mastering Python Pandas can transform your data science projects. This powerful library simplifies complex data tasks. Start exploring its features today to elevate your data analysis skills. With practice, you’ll become proficient and efficient, unlocking new insights in your data.
Happy coding with Python Pandas!